月末日を取得する方法
calendar.monthrange() メソッドに年と月を渡すと、月初日の曜日(0:月曜~6:日曜)と、その月が何日あるかをTupleで返します。import calendar
Tuple(0:月曜~6:日曜, 月の日数) = datetimeオブジェクト.date(年, 月)
# 月末日だけで良い場合はこちら
月の日数 = datetimeオブジェクト.date(年, 月)[1]
次のサンプルコードは 2099年12月の最終日を表示します。
import calendar
# 2099年12月の最終日を取得
lastDay = calendar.monthrange(2099,12)[1]
# 結果 = 2099年12月の最終日は31日
print(f'2099年12月の最終日は{lastDay}日')
今月の月末日を取得する
datetime.date.today() メソッドを使用すると システム年月日 を date型 で返します。date型 の yearプロパティ と monthプロパティ で年月が得られるので、calendar.monthrange()メソッドの引数に渡します。次のサンプルコードはシステム年月の最終日を表示します。
import calendar
import datetime
# システム年月日を取得
today = datetime.date.today()
# システム年月の最終日を取得
lastDay = calendar.monthrange(today.year, today.month)[1]
# 結果 = 2022年11月の最終日は30日
print(f'{today.year}年{today.month}月の最終日は{lastDay}日')
システム年月(今月)の月末日をdate型で取得
date型 のコンストラクタは date(年, 月, 日付) となっているので、日付に calendar.monthrange()メソッド で得られた月末日を渡します。次のサンプルコードはシステム年月の最終日をdate型にしています。
import calendar
import datetime
# システム年月日を取得
today = datetime.date.today()
# システム年月の最終日を取得
lastDay = calendar.monthrange(today.year, today.month)[1]
# date型 にする
lastDate = datetime.date(today.year, today.month, lastDay)
# 結果 = 2022-11-30の型は<class 'datetime.date'>
print(f'{lastDate}の型は{type(lastDate)}')
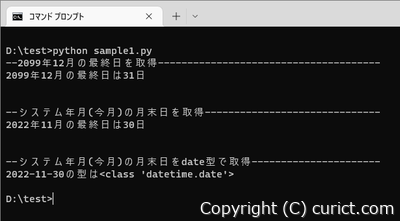
参考資料
検証環境
- Python 3.11.0 (main, Oct 24 2022, 18:26:48) [MSC v.1933 64 bit (AMD64)] on win32
- Microsoft Windows 10 Enterprise Version 21H2 OS Build 19044.2130 Experience: Windows Feature Experience Pack 120.2212.4180.0