フォルダ(ディレクトリ)があるか、確認する方法
os.path.isdir()メソッドの引数に確認したいファイルのパスを渡します。フォルダがあれば True を返し、それ以外は False を返します。os.path.isdir の使用方法
import os
結果(True or False) = os.path.isdir('ファイルパス')
- 引数に渡したフォルダ(ディレクトリ)があると True、それ以外は False を返します。
- ファイルの場合、存在しても False を返します。ファイルの存在確認には「os.path.isfile」を使用します。
- シンボリックリンクの場合、リンク先を辿って判定します。シンボリックリンクかを確認するには「os.path.islink」を使用します。(Windowsのショートカットの場合、リンク先ファイルがあっても False を返します。)
- Pythonバージョン3.6から、引数に文字列以外にも path-like object(pathlibなど) を渡せるようになりました。
サンプルコード1
次のサンプルコードは、フォルダ「test-dir」があるか確認しています。import os
# ディレクトリがあるか判定
filePath = './test-dir'
result = os.path.isdir(filePath)
if result:
print(f'ディレクトリ{filePath}は存在します。')
else:
print(f'ディレクトリ{filePath}は存在しません。')
サンプルコード2
フォルダ(ディレクトリ)やショートカット(Windows)の有無を確認しようとしています。import os
# ディレクトリがある場合、True を返す
filePath = './test-dir'
result = os.path.isdir(filePath)
if result:
print(f'ディレクトリ{filePath}は存在します。')
else:
print(f'ディレクトリ{filePath}は存在しません。')
# ファイルの場合は存在していても False を返す
filePath = './test.txt'
result = os.path.isdir(filePath)
if result:
print(f'ディレクトリ{filePath}は存在します。')
else:
print(f'ディレクトリ{filePath}は存在しません。')
# ショートカット(Windows)の場合は False を返す
filePath = './test-dir - ショートカット'
result = os.path.isdir(filePath)
if result:
print(f'ディレクトリ{filePath}は存在します。')
else:
print(f'ディレクトリ{filePath}は存在しません。')
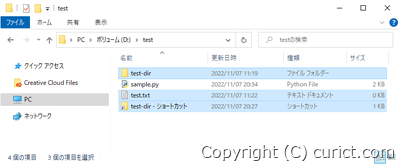
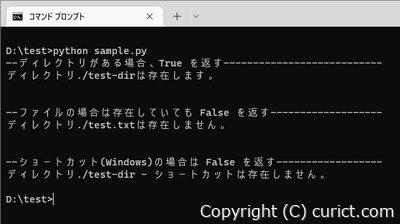
検証環境
- Python 3.11.0 (main, Oct 24 2022, 18:26:48) [MSC v.1933 64 bit (AMD64)] on win32
- Microsoft Windows 10 Pro Version 21H2 OS Build 19044.2130 Experience: Windows Feature Experience Pack 120.2212.4180.0